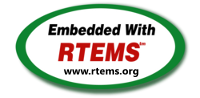 |
RTEMS
5.0.0
|
Go to the documentation of this file. 61 #ifndef __USER_LABEL_PREFIX__ 62 #define __USER_LABEL_PREFIX__ 65 #ifndef __REGISTER_PREFIX__ 66 #define __REGISTER_PREFIX__ "$" 73 #define SYM(x) CONCAT1 (__USER_LABEL_PREFIX__, x) 77 #define REG(x) CONCAT1 (__REGISTER_PREFIX__, x) 106 #define BEGIN_CODE_DCL .text 108 #define BEGIN_DATA_DCL .data 110 #define BEGIN_CODE asm ( ".text 123 #define PUBLIC(sym) .globl SYM (sym) 124 #define EXTERN(sym) .globl SYM (sym)