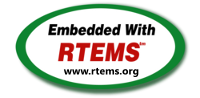 |
RTEMS
5.0.0
|
Go to the documentation of this file. 46 #define COLOR_BLACK 0x000000 47 #define COLOR_WHITE 0xFFFFFF 49 #define COLOR_BLUE 0x0000FF 50 #define COLOR_GREEN 0x00FF00 51 #define COLOR_RED 0xFF0000 53 #define COLOR_NAVY 0x000080 54 #define COLOR_DARKBLUE 0x00008B 55 #define COLOR_DARKGREEN 0x006400 56 #define COLOR_DARKCYAN 0x008B8B 57 #define COLOR_CYAN 0x00FFFF 58 #define COLOR_TURQUOISE 0x40E0D0 59 #define COLOR_INDIGO 0x4B0082 60 #define COLOR_DARKRED 0x800000 61 #define COLOR_OLIVE 0x808000 62 #define COLOR_GRAY 0x808080 63 #define COLOR_SKYBLUE 0x87CEEB 64 #define COLOR_BLUEVIOLET 0x8A2BE2 65 #define COLOR_LIGHTGREEN 0x90EE90 66 #define COLOR_DARKVIOLET 0x9400D3 67 #define COLOR_YELLOWGREEN 0x9ACD32 68 #define COLOR_BROWN 0xA52A2A 69 #define COLOR_DARKGRAY 0xA9A9A9 70 #define COLOR_SIENNA 0xA0522D 71 #define COLOR_LIGHTBLUE 0xADD8E6 72 #define COLOR_GREENYELLOW 0xADFF2F 73 #define COLOR_SILVER 0xC0C0C0 74 #define COLOR_LIGHTGREY 0xD3D3D3 75 #define COLOR_LIGHTCYAN 0xE0FFFF 76 #define COLOR_VIOLET 0xEE82EE 77 #define COLOR_AZUR 0xF0FFFF 78 #define COLOR_BEIGE 0xF5F5DC 79 #define COLOR_MAGENTA 0xFF00FF 80 #define COLOR_TOMATO 0xFF6347 81 #define COLOR_GOLD 0xFFD700 82 #define COLOR_ORANGE 0xFFA500 83 #define COLOR_SNOW 0xFFFAFA 84 #define COLOR_YELLOW 0xFFFF00 93 #define BLUE_LEV(level) ((level)&BLUE) 94 #define GREEN_LEV(level) ((((level)*2)<<5)&GREEN) 95 #define RED_LEV(level) (((level)<<(5+6))&RED) 96 #define GRAY_LEV(level) (BLUE_LEV(level) | GREEN_LEV(level) | RED_LEV(level)) 98 #define RGB_24_TO_RGB565(RGB) \ 99 (((RGB >>19)<<11) | (((RGB & 0x00FC00) >>5)) | (RGB & 0x00001F)) 100 #define RGB_24_TO_18BIT(RGB) \ 101 (((RGB >>16)&0xFC) | (((RGB & 0x00FF00) >>10) << 10) | (RGB & 0x0000FC)<<16) 102 #define RGB_16_TO_18BIT(RGB) \ 103 (((((RGB >>11)*63)/31)<<18) | (RGB & 0x00FC00) | (((RGB & 0x00001F)*63)/31)) 104 #define BGR_TO_RGB_18BIT(RGB) \ 105 (RGB & 0xFF0000) | ((RGB & 0x00FF00) >> 8) | ((RGB & 0x0000FC) >> 16)) 106 #define BGR_16_TO_18BITRGB(RGB) BGR_TO_RGB_18BIT(RGB_16_TO_18BIT(RGB))