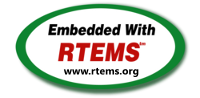 |
RTEMS
5.0.0
|
Go to the documentation of this file. 19 #ifndef __AT91RM9200_EMAC_H__ 20 #define __AT91RM9200_EMAC_H__ 31 #define EMAC_RBQP 0x18 39 #define EMAC_SCOL 0x44 40 #define EMAC_MCOL 0x48 42 #define EMAC_SEQE 0x50 45 #define EMAC_LCOL 0x5C 46 #define EMAC_ECOL 0x60 53 #define EMAC_SQEE 0x7C 54 #define EMAC_DRFC 0x80 57 #define EMAC_SA1L 0x98 58 #define EMAC_SA1H 0x9C 59 #define EMAC_SA2L 0xA0 60 #define EMAC_SA2H 0xA4 61 #define EMAC_SA3L 0xA8 62 #define EMAC_SA3H 0xAC 63 #define EMAC_SA4L 0xB0 64 #define EMAC_SA4H 0xB4 67 #define EMAC_CTL_LB BIT0 68 #define EMAC_CTL_LBL BIT1 69 #define EMAC_CTL_RE BIT2 70 #define EMAC_CTL_TE BIT3 71 #define EMAC_CTL_MPE BIT4 72 #define EMAC_CTL_CSR BIT5 73 #define EMAC_CTL_ISR BIT6 74 #define EMAC_CTL_WES BIT7 75 #define EMAC_CTL_BP BIT8 78 #define EMAC_CFG_SPD BIT0 79 #define EMAC_CFG_FD BIT1 80 #define EMAC_CFG_BR BIT2 81 #define EMAC_CFG_CAF BIT4 82 #define EMAC_CFG_NBC BIT5 83 #define EMAC_CFG_MTI BIT6 84 #define EMAC_CFG_UNI BIT7 85 #define EMAC_CFG_BIG BIT8 86 #define EMAC_CFG_EAE BIT9 87 #define EMAC_CFG_CLK_8 (0 << 10) 88 #define EMAC_CFG_CLK_16 (1 << 10) 89 #define EMAC_CFG_CLK_32 (2 << 10) 90 #define EMAC_CFG_CLK_64 (3 << 10) 91 #define EMAC_CFG_CLK_MASK (3 << 10) 92 #define EMAC_CFG_RTY BIT12 93 #define EMAC_CFG_RMII BIT13 96 #define EMAC_LINK BIT0 97 #define EMAC_MDIO BIT1 98 #define EMAC_IDLE BIT2 101 #define EMAC_TCR_LEN(_x_) ((_x_ & 0x7FF) << 0) 102 #define EMAC_TCR_NCRC BIT15 105 #define EMAC_TSR_OVR BIT0 106 #define EMAC_TSR_COL BIT1 107 #define EMAC_TSR_RLE BIT2 108 #define EMAC_TSR_TXIDLE BIT3 109 #define EMAC_TSR_BNQ BIT4 110 #define EMAC_TSR_COMP BIT5 111 #define EMAC_TSR_UND BIT6 114 #define EMAC_RSR_BNA BIT0 115 #define EMAC_RSR_REC BIT1 116 #define EMAC_RSR_OVR BIT2 124 #define EMAC_INT_DONE BIT0 125 #define EMAC_INT_RCOM BIT1 126 #define EMAC_INT_RBNA BIT2 127 #define EMAC_INT_TOVR BIT3 128 #define EMAC_INT_TUND BIT4 129 #define EMAC_INT_RTRY BIT5 130 #define EMAC_INT_TBRE BIT6 131 #define EMAC_INT_TCOM BIT7 132 #define EMAC_INT_TIDLE BIT8 133 #define EMAC_INT_LINK BIT9 134 #define EMAC_INT_ROVR BIT10 135 #define EMAC_INT_ABT BIT11 138 #define EMAC_MAN_DATA(_x_) ((_x_ & 0xFFFF) << 0) 139 #define EMAC_MAN_CODE (0x2 << 16) 140 #define EMAC_MAN_REGA(_x_) ((_x_ & 0x1F) << 18) 141 #define EMAC_MAN_PHYA(_x_) ((_x_ & 0x1F) << 23) 142 #define EMAC_MAN_WRITE (0x1 << 28) 143 #define EMAC_MAN_READ (0x2 << 28) 144 #define EMAC_MAN_HIGH BIT30 145 #define EMAC_MAN_LOW BIT31 151 #define RXBUF_ADD_BASE_MASK 0xfffffffc 152 #define RXBUF_ADD_WRAP BIT1 153 #define RXBUF_ADD_OWNED BIT0 156 #define RXBUF_STAT_BCAST BIT31 157 #define RXBUF_STAT_MULTI BIT30 158 #define RXBUF_STAT_UNI BIT29 159 #define RXBUF_STAT_EXT BIT28 160 #define RXBUF_STAT_UNK BIT27 161 #define RXBUF_STAT_LOC1 BIT26 162 #define RXBUF_STAT_LOC2 BIT25 163 #define RXBUF_STAT_LOC3 BIT24 164 #define RXBUF_STAT_LOC4 BIT23 165 #define RXBUF_STAT_LEN_MASK 0x7ff