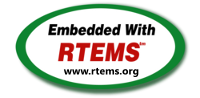 |
RTEMS
5.0.0
|
Go to the documentation of this file. 22 #include <sys/cdefs.h> 39 #if defined(_POSIX_ASYNCHRONOUS_IO) 46 #include <sys/types.h> 55 #define AIO_CANCELED 0 56 #define AIO_NOTCANCELED 1 88 volatile void *aio_buf;
91 struct sigevent aio_sigevent;
120 struct aiocb *__restrict
const list[__restrict],
122 struct sigevent *__restrict sig
130 const struct aiocb *aiocbp
139 const struct aiocb *aiocbp
166 const struct aiocb *
const list[],
168 const struct timespec *timeout
171 #if defined(_POSIX_SYNCHRONIZED_IO)